Managing Multiple Node Versions
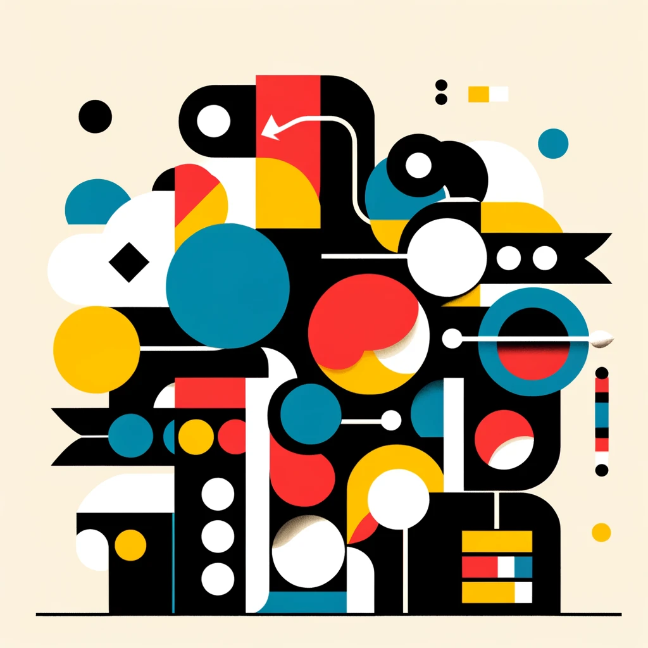
I have a few projects on my local machine which require specific versions of Node. I learned today how I can have multiple versions of Node installed and automatically configure a project to use a specific version when I switch to its directory.
Install Node Version Manager (NVM)
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
After installing, restart the terminal or source your profile script to ensure NVM is loaded:
export NVM_DIR="$HOME/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm
Install multiple versions of Node
nvm install 18.12.1 # Install Node.js version 18.12.1
nvm install 20.12.1 # Install Node.js version 20.12.1
To manually switch between multiple versions of Node, run nvm use
:
nvm use 18.12.1 # Switch to Node.js version 18.12.1
nvm use 20.12.1 # Switch to Node.js version 20.12.1
To automate Node version switching for specific projects
First, place a .nvmrc
file in the root of the project directory with the required Node version noted inside:
echo "18.12.1" > .nvmrc
Then, to automate the process of switching Node versions based on the .nvmrc
file when you cd
into that root directory, you can add the following function to your shell's configuration file (.bashrc, .bash_profile, .zshrc, etc.):
autoload -U add-zsh-hook
load-nvmrc() {
local node_version="$(nvm version)"
local nvmrc_path="$(nvm_find_nvmrc)"
if [ -n "$nvmrc_path" ]; then
local nvmrc_node_version=$(nvm version "$(cat "${nvmrc_path}")")
if [ "$nvmrc_node_version" = "N/A" ]; then
nvm install
elif [ "$nvmrc_node_version" != "$node_version" ]; then
nvm use
fi
elif [ "$node_version" != "$(nvm version default)" ]; then
echo "Reverting to default node version"
nvm use default
fi
}
add-zsh-hook chpwd load-nvmrc
load-nvmrc
This script does the following:
- Checks if there's an
.nvmrc
file in the current directory. - If there is, it switches to the Node version specified in that file.
- Upon leaving the directory, it switches back to the default Node version.
Apply Changes to your shell configuration
source ~/.zshrc